Over the last few months I have had the ability to mess around with a bit of jQuery. Even though I don’t have the complete understanding on how it works, I can see the benefits of writing my code in jQuery compared to bashing out lots of lines of JavaScript to do the same thing.
One the cool features I have used is calling one of my .NET methods using the “$.ajax” jQuery command. In my example (below), I have created two aspx pages. The code-behind of my first page (jQueryMethodTest.aspx) will only contain a public static method called “WhatIsYourName”, which returns a string value.
[WebMethod]
public static string WhatIsYourName(string name)
{
if (!String.IsNullOrEmpty(name))
{
return String.Concat("Hello ", name, "!");
}
else
{
return String.Empty;
}
}
Remember, the jQueryMethodTest.aspx page only needs to contain our method nothing else! Additional methods can be added. Just don’t add any web controls.
The second page (jQueryAjax.aspx), will contain our jQuery code and some HTML to output our result from calling the “WhatIsYourName” method.
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript" src="javascript/jquery.js"></script>
</head>
<script type="text/javascript">
$(document).ready(function() {
$("#btnSubmitName").click(function(event) {
$.ajax({
type: "POST",
url: "jQueryMethodTest.aspx/WhatIsYourName",
data: "{'name': '" + $('#name').val() + "'}",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(message) {
ShowPopup(message);
},
error: NameFailed
});
});
});
function ShowPopup(result) {
if (result.d != "") {
$("#Message").html(result.d);
}
else {
$("#Message").html("I didn't get your name.");
}
}
function NameFailed(result) {
$("#Message").html(result.status + ' ' + result.statusText);
}
</script>
<body>
<form id="form1" runat="server">
<div>
<input id="name" name="name" type="text" />
<br />
<input id="btnSubmitName" name="btnSubmitName" type="button" value="Submit" />
<br /><br />
<span id="Message" style="color:Red;"></span>
</div>
</form>
</body>
</html>
If all goes well, you should get the following result:
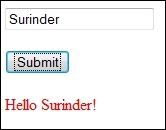
The “$.ajax” jQuery command requires the following parameters in order to work:
- url – links to where our .NET method is placed.
- data – retrieves the value from some control in our page to pass to our method. Remember, the name of the parameter must be named the same as the parameter from our .NET method.
- dataType – the response type.
- contentType – the request content type.
- success – the JavaScript function that gets fired on postback.
- error – the Javascript function that gets fired if there is a failure. This is an optional parameter.
I guess jQuery’s motto really is true: “write less, do more”.