C# Variable Type: To 'var', Or Not To 'var'
This post has been in the works for some time in order to express my annoyance whenever I see every variable in a C# project declared with "var". This is simply laziness... I guess typing three letters is simpler than typing the actual variable type. Even so, I believe that this, among other things, contributes to readability issues.
I am not completely opposed to its use, as I use it in places where I deem it acceptable and has no effect on readability. My biggest gripe is when an entire project is littered solely with this form of variable declaration. Whenever I come across a project like this, I have to run a Visual Studio tool that automatically changes variables to use an explicit type instead.
Some may argue that readability is unaffected because you can obtain type information simply by hovering over any variable in Visual Studio. A perfectly valid point. However, things become more obfuscated when quickly glancing at some code via Notepad, Github.com, or during code reviews.
From what I've seen on forums, there are various points of view, some of which I agree with and wanted to share my thoughts.
Opinion 1: Saves Time Through Less Keystrokes
List<ContactInfoProvider> contacts = new List<ContactInfoProvider>();
// vs.
var contacts = new List<ContactInfoProvider>();
This assumes a developer is using an IDE with no intellisense capability. This shouldn't be a problem since intellisense will quickly resolve the class and types in use. According to some developers I work with, declaring long type names is messy and adds unnecessary extra noise.
I don't mind this approach since the type in use is evident.
Opinion 2: Readability Is Not An Issue
To expand upon the point I made in my intro about code being obfuscated, lets take the following snippet of code:
...
var contactInfo = ContactInfoProvider.GetContactInfo(token);
...
I'm unable to see what type is being returned immediately unless I delve into the ContactInfoProvider
class and view the method. "var" should never be used where the type is not known because it reduces recognition readability in code.
Opinion 3: Use "var" On Basic Variable Types
var maxRecords = 100;
var price = 1.1;
var successMessage = "Surinder thinks this is wrong!";
var isSuccess = false;
// vs.
int maxRecords = 100;
decimal price = 1.1;
string successMessage = "Surinder thinks this is correct!";
bool isSuccess = true;
I did state earlier the use of "var" is acceptable where the type used is clearly visible. Basic variable types is an exception to the rule as it's completely unnecessary.
Opinion 4: Encourages Descriptive Variable Names
var productItem = new ProductInfo()
// vs.
ProductInfo pi = new ProductInfo()
This is where I disagree. Descriptive variable names should always be used, regardless of whether the variable is declared explicitly or implicitly. In my time as a developer, I've seen some dreadful variable names in both instances.
Conclusion
There is no right or wrong way to use "var," and it is entirely up to personal preference. I only use "var" when I'm writing quick test code, playing with LINQ, or when I'm not sure what the outcome of a method is when dealing with external API libraries.
Even though I relish learning new code notations and refactoring, I will continue to use "var" very sparingly (if at all!) in my own code. But the overall use of it just doesn't sit right with me. Strict typing ensures the most clearest approach, so a developer knows exactly what is going to happen once it is typed.
We should always aim to make our code as clear as possible. It's difficult enough to understand explicitly set variables that aren't self-descriptive without having to add "var" to the mix.
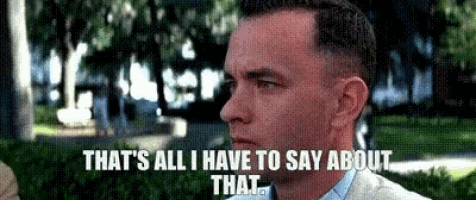