I have noticed that one of many reasons clients like to get on to the SharePoint bandwagon is to use its detailed Document Management features to control the life cycle of each individual document within their organisation.
This got me thinking. Most organisations have hundreds, if not thousands of documents they would like to move from their networked storage devices to the SharePoint 2007 platform. It would be time consuming to upload all these documents to a document library. So I decided to create a C# application that would allow me to upload multiple files from a folder on a PC to a document library web part of my choice.
Just to note, this program I created has not been tested to upload documents in their thousands. I have tested uploading over 100 documents successfully. But feel free to modify my code to work more efficiently! ;-)

As you can see from my program above, I have managed to upload numerous documents from “Z:\My Received Files” to a document library called “Shared Documents”.
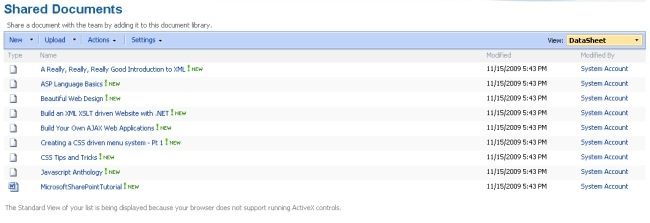
I created my program by using the following code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using Microsoft.SharePoint;
using System.IO;
namespace MOSSDocumentLibraryUploader
{
public partial class frmHome : Form
{
public frmHome()
{
InitializeComponent();
}
private void frmHome_Load(object sender, EventArgs e)
{
ddlDocumentLibList.Enabled = false;
ddlSubSites.Enabled = false;
}
private void btnStartUpload_Click(object sender, EventArgs e)
{
lstUploadedDocuments.Items.Add(String.Format("Upload operation started at {0} {1}", DateTime.Now.ToShortDateString(), DateTime.Now.ToLongTimeString()));
//Start uploading files
try
{
//Get site collection, website and document library informationn
SPSite intranetSite = new SPSite(txtIntranetUrl.Text);
SPWeb intranetWeb = intranetSite.AllWebs[ddlSubSites.SelectedItem.ToString()];
SPList documentLibrary = intranetWeb.Lists[ddlDocumentLibList.SelectedItem.ToString()];
intranetWeb.AllowUnsafeUpdates = true;
intranetWeb.Lists.IncludeRootFolder = true;
//Start iterating through all files in you local directory
string[] fileEntries = Directory.GetFiles(txtDocumentDirectory.Text);
foreach (string filePath in fileEntries)
{
SPFile file;
//Get file information
FileInfo fInfo = new FileInfo(filePath);
Stream fileStream = new FileStream(filePath, FileMode.Open);
//Load contents into a byte array
Byte[] contents = new Byte[fInfo.Length];
fileStream.Read(contents, 0, (int)fInfo.Length);
fileStream.Close();
//Upload file to SharePoint Document library
file = intranetWeb.Files.Add(String.Format("{0}/{1}/{2}", intranetWeb.Url, documentLibrary.Title, fInfo.Name), contents);
file.Update();
lstUploadedDocuments.Items.Add(String.Format("Successfully uploaded: {0}", fInfo.Name));
lstUploadedDocuments.Refresh();
}
//Perform clean up
intranetWeb.Dispose();
intranetSite.Dispose();
lstUploadedDocuments.Items.Add(String.Format("Operation completed at {0}", DateTime.Now.ToLongTimeString()));
}
catch (Exception ex)
{
lstUploadedDocuments.Items.Add(String.Format("Error: {0}", ex));
}
}
private void btnCancelUpload_Click(object sender, EventArgs e)
{
lstUploadedDocuments.Items.Add(String.Format("Upload operation cancelled at {0} {1}", DateTime.Now.ToShortDateString(), DateTime.Now.ToLongTimeString()));
}
private void txtDocumentDirectory_Validating(object sender, CancelEventArgs e)
{
if (((TextBox)sender).Text == "")
{
MessageBox.Show("You must enter an upload directory.", "Invalid Input", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
else if (!Directory.Exists(((TextBox)sender).Text))
{
MessageBox.Show("Document upload directory does not exist.", "Directory Does Not Exist", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
private void txtIntranetUrl_Validating(object sender, CancelEventArgs e)
{
if (((TextBox)sender).Text == "")
{
MessageBox.Show("You must enter a intranet url.", "Invalid Input", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
}
private void btnExit_Click(object sender, EventArgs e)
{
this.Close();
}
private void ddlSubSites_SelectedIndexChanged(object sender, EventArgs e)
{
try
{
ddlDocumentLibList.Enabled = true;
//Get site collection, website and document library informationn
SPSite intranetSite = new SPSite(txtIntranetUrl.Text);
SPWeb intranetWeb = intranetSite.AllWebs[ddlSubSites.SelectedItem.ToString()];
intranetWeb.Lists.IncludeRootFolder = true;
//Iterate through all document libraries and populate ddlDocumentLibList
foreach (SPList docList in intranetWeb.Lists)
{
ddlDocumentLibList.Items.Add(docList.Title);
}
}
catch
{
ddlDocumentLibList.Enabled = false;
}
}
private void txtIntranetUrl_TextChanged(object sender, EventArgs e)
{
try
{
ddlSubSites.Enabled = true;
ddlDocumentLibList.Enabled = true;
//Get site collection, website and document library information
SPSite intranetSite = new SPSite(txtIntranetUrl.Text);
//Iterate through all sites and propulate ddlSubSites
foreach (SPWeb web in intranetSite.AllWebs)
{
ddlSubSites.Items.Add(web.Url.Replace(txtIntranetUrl.Text, ""));
//Iterate through child sites
foreach (SPWeb childSite in web.Webs)
{
ddlSubSites.Items.Add(childSite.Url.Replace(txtIntranetUrl.Text, ""));
}
}
}
catch
{
ddlSubSites.Enabled = false;
ddlDocumentLibList.Enabled = false;
}
}
}
}
It would have been really cool if my program only listed Document Libraries instead of all lists within a portal site. Unfortunately, I could not find any code to get a list of type Document Library. If anyone knows how to do this, I would be grateful if you could post some code.
If you have any questions on the code or know of a better (free) solution out there, please leave a comment.